最近はPHPをやってきたのですが、Python3をやる必要が出てきました。
基礎はざっとやった上で、今の所の基礎が詰まっていたコードを読み解いたので記事にします。
ソースコードについて
今回取り上げるソースコードは、
テキストで用意した、生徒の各教科の点数が書いてあるpoint.txtというファイルを読み込み、
整形して、合計点、平均点、合計点に対する評価(ExcellentやBadなど)を行い、evaluation.txtというファイルで出力させるプログラム(evaluate.py)です。
evaluate.py
# point.txt を開き、open_fileに代入
open_file = open('point.txt')
# 開いたopen_fileを読む。それをraw_dataに代入。
raw_data = open_file.read()
# 開いたopen_fileを閉じる。
open_file.close()
# 行ごとのリストへ変換
# splitlines()関数は、文字列を改行ごとに区切り、リスト型で返す。
point_data = raw_data.splitlines()
# データ格納用の辞書作成(まずは空っぽ)
point_dict = {}
# 繰り返し文 point_dataのキーをlineにコピー
for line in point_data:
# コロンで文字列を「氏名」と「複数の点数」に分割
student_name, points_str = line.split(':')
# point_dict辞書に student_nameをキーとして points_strを登録
point_dict[student_name] = points_str
# 合計点と平均点の辞書作成(まずは空っぽ)
score_dict = {}
# point_dict を student_nameにコピーして繰り返し処理開始
for student_name in point_dict:
# 点数リストを作りたい
# → point_dictの student_nameをキーとして、取得した点数の文字列をカンマで区切ってpoint_listに代入。
point_list = point_dict[student_name].split(',')
# point_listの要素の数を取得し、subject_numberに代入。
subject_number = len(point_list)
# 合計値を示す変数 total は最初(初期値)は 0とする。
total = 0
# point_list をpointにコピーして繰り返し処理開始
for point in point_list:
# 合計を求める。 今までのtotal に、 int型に型変換したもともとstr型のpointを足す。
total = total + int(point)
# 平均を求める。 合計点を教科数で割れば平均が出る。
average = total / subject_number
# score_dict辞書に student_nameをキーとしてデータを登録。
# 0番目が total 1番目が average 2番目が subject_number
score_dict[student_name] = (total, average, subject_number)
# 評価結果のための辞書を作成。
evaluation_dict = {}
# score_dictをstudent_nameにコピーして繰り返し処理開始
for student_name in score_dict:
# score_dictを student_nameをキーとして score_dataに代入。
score_data = score_dict[student_name]
# score_dataの0番目を取り出し、totalに代入
total = score_data[0]
# score_dataの1番目を取り出し、averageに代入
average = score_data[1]
# score_dataの2番目を取り出し、score_dataに代入
subject_number = score_data[2]
# subject_number (教科数) × 100(点) × 0.8(80%)のときはexcellentに代入
excellent = subject_number * 100 * 0.8
# subject_number (教科数) × 100(点) × 0.65(65%)のときはgoodに代入
good = subject_number * 100 * 0.65
# 上記を踏まえて if 文
if total >= excellent:
evaluation = 'Excellent!!!'
elif total >= good:
evaluation = 'Good!!'
else:
evaluation = 'Bad!'
# evaluationの値を evaluation_dict辞書に student_nameをキーとして登録
evaluation_dict[student_name] = evaluation
# 計算と評価をしたデータをアウトプットする
# evaluation.txtというファイルを開く(存在しない場合は作る。)
file_name = 'evaluation.txt'
# file_nameに対して、書き込みを行い、output_fileに代入
# 大抵、 open(filename, mode) のように二つの引数を伴って呼び出されます。
output_file = open(file_name, 'w')
# score_dict を student_name にコピーして繰り返し処理開始
for student_name in score_dict:
# score_dict を student_nameをキーにして取得し、 score_dataに代入
score_data = score_dict[student_name]
# 合計を出すのは、score_dataの0番目。(もともとscore_dict)それをtotalに代入。
total = score_data[0]
# evaluation_dict辞書から、student_nemeをキーとして取得し、 evaluationに代入
# => 評価を取り出している。
evaluation = evaluation_dict[student_name]
# 結果を書き込み、textに代入。
# {}の部分は、format()にある順番に 1つめstudent_name, 2つめtotal, 3つめevaluation
text = '[{}] total: {}, evaluation: {}\n'.format(student_name, total, evaluation)
#
output_file.write(text)
# 使った output_file ファイルを閉じる。
output_file.close()
# 最後に結果を出力。
print('評価結果を{}に出力しました。'.format(file_name))
point.txt
最初にopenしてreadしているテキストファイルはこちらです。
Json Pee:99,32,80
Andrew Core:90,80,79
Dame Omae:3,28,26
Python3 実行
実行してみます。

すると、最終行に記載した
print(‘評価結果を{}に出力しました。’.format(file_name))
が出力されているのがわかります。
evaluation.txt
最終的にアウトプットされるテキストファイルはこちらです。
[Json Pee] total: 211, evaluation: Good!!
[Andrew Core] total: 249, evaluation: Excellent!!!
[Dame Omae] total: 57, evaluation: Bad!
参考資料
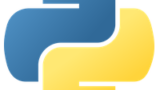
Python チュートリアル
Python は強力で、学びやすいプログラミング言語です。効率的な高レベルデータ構造と、シンプルで効果的なオブジェクト指向プログラミング機構を備えています。 Python は、洗練された文法・動的なデータ型付け・インタープリタであることなどから、スクリプティングや高速アプリケーション開発(Rapid Applicati...
はじめに — pep8-ja 1.0 ドキュメント